Example: Boost ptr_list:
01 | #include <boost/ptr_container/ptr_list.hpp> |
15 | boost::ptr_list<ABC> intList; |
16 | boost::ptr_list<ABC>::iterator iterIntList; |
30 | for (iterIntList = intList.begin(); |
31 | iterIntList != intList.end(); |
34 | cout << iterIntList->i << endl; |
Compile: g++ testBoostPtrList.cpp
Run: ./a.out
1
2
3
See
http://www.boost.org/libs/ptr_container/doc/ptr_list.html
Red Hat RPM packages: boost, boost-devel
Newer Linux releases like Red Hat Enterprise 5/CentOS 5 include boost "ptr_list".
RHEL4 included boost libraries but did not include "ptr_list".
Ubuntu installation: apt-get install libboost-dev libboost-doc
Example: STL list of pointers:
17 | list<ABC*>::iterator iterIntList; |
31 | for (iterIntList = intList.begin(); |
32 | iterIntList != intList.end(); |
35 | cout << (*iterIntList)->i << endl; |
39 | for (iterIntList = intList.begin(); |
40 | iterIntList != intList.end(); |
This example shows how one must delete each pointer individually.
For more information on STL list, see the YoLinux.com STL vector and STL list tutorial
GDB: pointers and memory investigation
You can test the above programs and investigate their respective memory
clean-up in GDB.
See the YoLinux.com GDB tutorial.
Using GDB:
- Compile with debugging enabled: g++ -g -o testStlPtrList testStlPtrList.cpp
- Start GDB: gdb testStlPtrList
- Show listing with line numbers: list
- Set break point: break 30
- Run program till break point: run
- Find memory location of variable in GDB: print &(c->i)
(gdb) p &(c->i)
$4 = (int *) 0x503050
- Dereference memory location: p (*0x503050)
(gdb) p (*0x503050)
$5 = 3
- Dereference memory location after memory is freed: p (*0x503050)
(gdb) p (*0x503050)
$7 = 0
Using RHEL5. Note older systems may give you a nonsense value.

Books:
 |
The C++ Standard Library: A Tutorial Reference
Nicolai M. Josuttis
ISBN #0201379260, Addison Wesley Longman
This book is the only book I have seen which covers string classes as
implemented by current Linux distributions.
It also offers a fairly complete coverage of the C++ Standard Template
Library (STL). Good reference book.
|
|
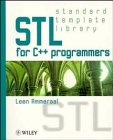 |
STL for C++ programmers
Leen Ammeraal
ISBN #0 471 97181 2, John Wiley & Sons Ltd.
Short book which teaches C++ Standard Template Library (STL) by example.
Not as great as a reference but is the best at introducing all the concepts
necessary to grasp STL completely and good if you want to learn STL quickly.
This book is easy to read and follow.
|
|
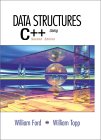 |
Data Structures with C++ Using STL
William Ford, Willaim Topp
ISBN #0130858501, Prentice Hall
|
|
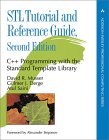 |
STL Tutorial and Reference Guide: C++ Programming with the Standard Template Library
David R. Musser, Gillmer J. Derge, Atul Saini
ISBN #0201379236, Addison-Wesley Publications
|
|
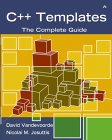 |
The C++ Templates: The complete guide.
David Vandevoorde, Nicolai Josuttis
ISBN #0201734842, Addison Wesley Pub Co.
Covers complex use of C++ Templates.
|
|
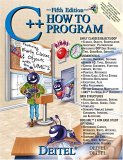 |
C++ How to Program
by Harvey M. Deitel, Paul J. Deitel
ISBN #0131857576, Prentice Hall
Fifth edition.
The first edition of this book (and Professor Sheely at UTA) taught me to
program C++. It is complete and covers all the nuances of the C++ language.
It also has good code examples. Good for both learning and reference.
|
|